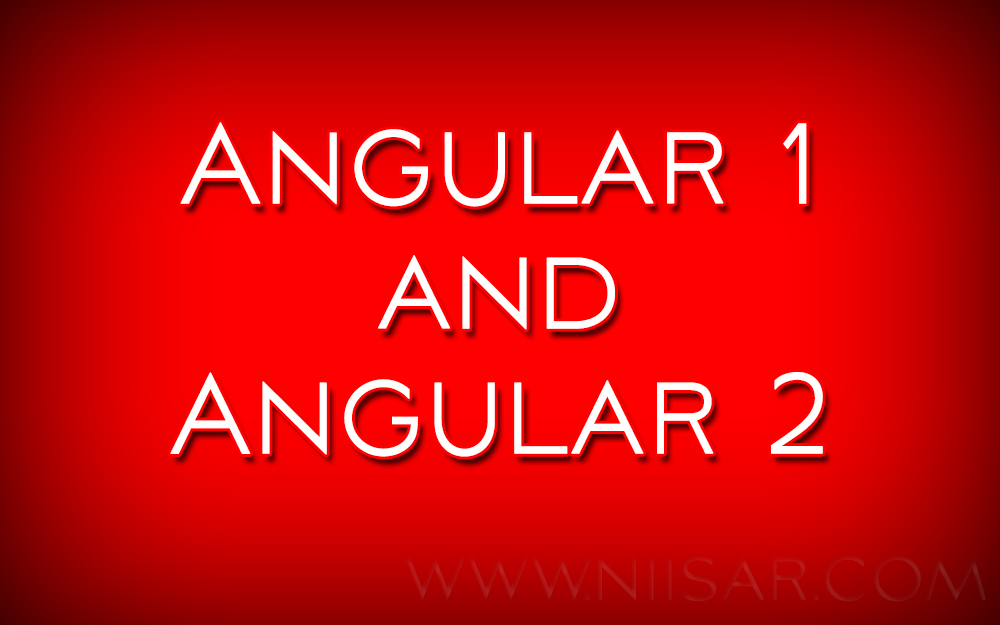
Comparing Component and Controller
The Component
Controllers are a big part of Angular 1 that is going away in Angular 2. In Angular 2 you will probably write all your controllers as components.
import {Component} from 'angular2/core' @Component({ selector: 'my-app', providers: [], template: ` <div> <h2>Hello {{name}}</h2> </div> `, directives: [] }) export class App { constructor() { this.name = 'Angular2' } }
<my-app> loading... </my-app>
The Controller
var app = angular.module('app', []); app.controller('MainCtrl', function($scope) { $scope.name = 'Hello Angular1'; });
<body ng-controller="MainCtrl"> <h2>{{name}}</h2> </body>
Structural Directives
*ngFor, *ngIf
<ul> <li *ngFor="#ball of balls"> {{ball.name}} </li> </ul> <div *ngIf="balls.length"> <h3>You have {{balls.length}} balls</h3> </div>
ng-repeat, ng-if
<ul> <li ng-repeat="ball in balls"> {{ball.name}} </li> </ul> <div ng-if="balls.length"> <h3>You have {{balls.length}} ball </h3> </div>
Two-Way Data Binding
[(ngModel)]='value'
<input [(ngModel)]="me.name">
ng-model='value'
<input ng-model="me.name">
Property Binding
[Property]='Property'
<div [style.visibility]="tools ? 'visible' : 'hidden'"> <img [src]="imagePath"> <a [href]="link">{{tools}}</a> </div>
ng-property='Property'
<div ng-style="tools ? {visibility: 'visible'}: {visibility: 'hidden'}"> <img ng-src="{{tools}}"> <a ng-href="{{tools}}"> {{tools}} </a> </div>
Event Binding
(event)='action()'
<input (blur)="log('blur')" (focus)="log('focus')" (keydown)="log('keydown', $event)" (keyup)="log('keyup', $event)" (keypress)="log('keypress', $event)" >
ng-event='action()'
<input ng-blur="log('blur')" ng-focus="log('focus')" ng-keydown="log('keydown', $event)" ng-keyup="log('keyup', $event)" ng-keypress="log('keypress', $event)" >
Services and DI
Injectable Service
In Angular 1 we use services by using any one of Factory
, Services
, Providers
, Constants
, Values
which all are covered under a provider.
But in Angular 2 all this are consolidated into one base Class
.
import {Injectable} from 'angular2/core'; @Injectable() export class StudentService { getStudents = () => [ { id: 1, name: 'Nisar' }, { id: 2, name: 'Sonu' }, { id: 3, name: 'Ram' } ]; }
Using same service in Component
import { Component } from 'angular2/core'; import { StudentService } from './student.service'; @Component({ selector: 'my-students', templateUrl: 'app/student.component.html', providers: [StudentService] }) export class StudentsComponent { constructor( private _StudentService: StudentService) { } students = this._StudentService.getStudents(); }
Service
(function () { angular .module('app') .service('StudentService', StudentService); function StudentService() { this.getStudents = function () { return [ { id: 1, name: 'X-Wing Fighter' }, { id: 2, name: 'Tie Fighter' }, { id: 3, name: 'Y-Wing Fighter' } ]; } } })();
Using same service in Controller
(function () { angular .module('app', []) .controller('StdController', StudentsController); StudentsController.$inject = ['StudentService']; function StdController(StudentService) { var std = this; std.title = 'Services'; std.Students = StudentService.getStudents(); } })();