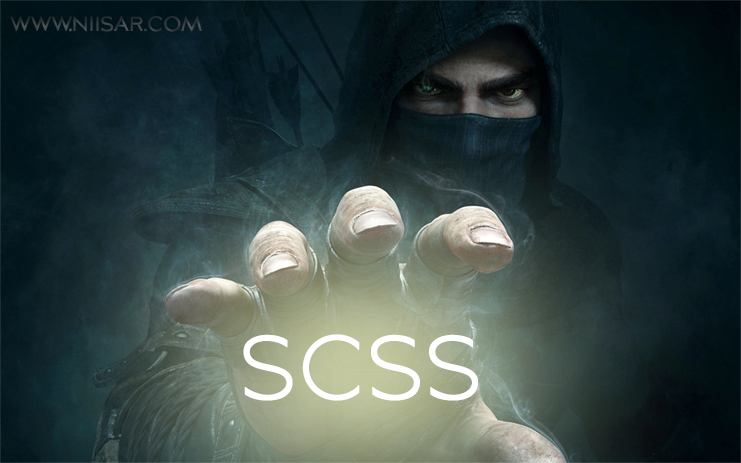
Nesting
SCSS
h1 { color: blue; span { color: gray; } }
CSS
h1 { color: blue; } h1 span { color: gray; }
Parent selector
SCSS
input{ border-color: gray; &:focus { border-color: orange; } }
CSS
input { border-color: gray; } input:focus { border-color: orange; }
Parent selector
SCSS
.advert button { font-size: 100%; .billboard @ { font-size: 200%; } }
CSS
.advert button { font-size: 100%; } .billboard .advert button { font-size: 200%; }
$ variables
SCSS
$color-main: rgb( 240, 139, 29 ); $color-accent: rgb( 141, 198, 63 ); h1 { color: $color-main; span { color: $color-accent; } }
CSS
h1 { color: #f08b1d; } h1 span { color: #8dc63f; }
Color helpers
SCSS
$main-color: rgb( 240, 139, 29 ); $main-color-light: lighten( $main-color, 30% ); $main-color-dark: darken( $main-color, 30% ); h1 { color: $color-main; span { color: $main-color-light; } span { color: $main-color-dark; } }
CSS
h1 { color: #f08b1d; } h1 span { color: #fad5ac; } h1 span { color: #6d3c07; }
@mixin and @include
SCSS
@mixin section-style { background-color: white; border-radius: 4px; box-shadow: 0 1px 3px rgba( 0, 0, 0, .7 ); } div { @include section-style; color:#rgb( 51, 51, 51 ); }
CSS
div { background-color: white; border-radius: 4px; box-shadow: 0 1px 3px rgba( 0, 0, 0, .7 ); color:#rgb( 51, 51, 51 ); }
Mixin variables
SCSS
@mixin border-radius( $radius ) { -webkit-border-radius: $radius; -moz-border-radius: $radius; border-radius: $radius; } div { @include border-radius( 4px ); }
CSS
div { -webkit-border-radius: 4px; -moz-border-radius: 4px; border-radius: 4px; }
Mixin variable defaults
SCSS
@mixin border-radius( $radius:10px ) { -webkit-border-radius: $radius; -moz-border-radius: $radius; border-radius: $radius; } div { @include border-radius( 4px ); } section { // no value here so default will be applied @include border-radius; }
CSS
div { -webkit-border-radius: 4px; -moz-border-radius: 4px; border-radius: 4px; } section { -webkit-border-radius: 10px; -moz-border-radius: 10px; border-radius: 10px; }
Mixins multiple variables
SCSS
@mixin box-shadow( $x:0, $y:0, $blur:4px, $spread:0, $color:black ) { -webkit-box-shadow: $x $y $blur $spread $color; -moz-box-shadow: $x $y $blur $spread $color; box-shadow: $x $y $blur $spread $color; } div { @include box-shadow( 0, 1, 10px, 0, rgba( 0, 0, 0, .7 ) ); } section { @include box-shadow( $blur:12px, $color:rgba( 0, 0, 0, .8 ) ); }
CSS
div { -webkit-box-shadow: 0 1 10px 0 rgba(0, 0, 0, 0.7); -moz-box-shadow: 0 1 10px 0 rgba(0, 0, 0, 0.7); box-shadow: 0 1 10px 0 rgba(0, 0, 0, 0.7); } section { -webkit-box-shadow: 0 0 12px 0 rgba(0, 0, 0, 0.8); -moz-box-shadow: 0 0 12px 0 rgba(0, 0, 0, 0.8); box-shadow: 0 0 12px 0 rgba(0, 0, 0, 0.8); }
Nested mixins
SCSS
@mixin border-radius( $radius:4px ) { -webkit-border-radius: $radius; -moz-border-radius: $radius; border-radius: $radius; } @mixin box-shadow( $x:0, $y:0, $blur:4px, $spread:0, $color:black ) { -webkit-box-shadow: $x $y $blur $spread $color; -moz-box-shadow: $x $y $blur $spread $color; box-shadow: $x $y $blur $spread $color; } @mixin div-style { background-color:white; @include border-radius( 6px ); @include box-shadow( $x:2, $blur:10px ); color:rgb( 51, 51, 51 ); } div { @include div-style }
CSS
div { background-color: white; -webkit-border-radius: 6px; -moz-border-radius: 6px; border-radius: 6px; -webkit-box-shadow: 2 0 10px 0 black; -moz-box-shadow: 2 0 10px 0 black; box-shadow: 2 0 10px 0 black; color: #333333; }
@extend
SCSS
div { background-color: white; border:1px solid gray; margin-bottom: 1rem; } section { @extend div; color: rgb( 102, 102, 102 ); }
CSS
div, section { background-color: white; border:1px solid gray; margin-bottom: 1rem; } section { color: #666666; }
Extending with %placeholders
SCSS
%default-text { font: { size: 100%; family: Georgia, "Times New Roman", serif; weight: normal; } line-height: 1.3; color: rgb( 51, 51, 51 ); } div { @extend %default-text; } section { @extend %default-text; } header { @extend %default-text; } aside { @extend %default-text; }
CSS
div, section, header ,aside { font-size: 100%; font-family: Georgia, "Times New Roman", serif; font-weight: normal; line-height: 1.3; color: rgb( 51, 51, 51 ); }
Nesting namespaced
SCSS
h1 { font: { family: Georgia, "Times New Roman", serif; size: 137.5%; weight: thin; } color: blue; }
CSS
h1 { font-family: Georgia, "Times New Roman", serif; font-size: 137.5%; font-weight :thin; color: blue; }
Expanded
SCSS
sass --watch --style expanded style.scss:style.css
CSS
ul { display: inline-block; } ul li { list-style: none; color: rgb(51,51,51); }
Compact
SCSS
sass --watch --style compact style.scss:style.css
CSS
ul { display: inline-block; } ul li { list-style: none; color: rgb(51,51,51); }
Compressed
SCSS
sass --watch --style compressed style.scss:style.css
CSS
ul{display:inline-block;}ul li{list-style:none;color:rgb(51,51,51);}
No comments:
Post a Comment